Top 5 Beginner-Friendly JavaScript Projects to Build in 2025
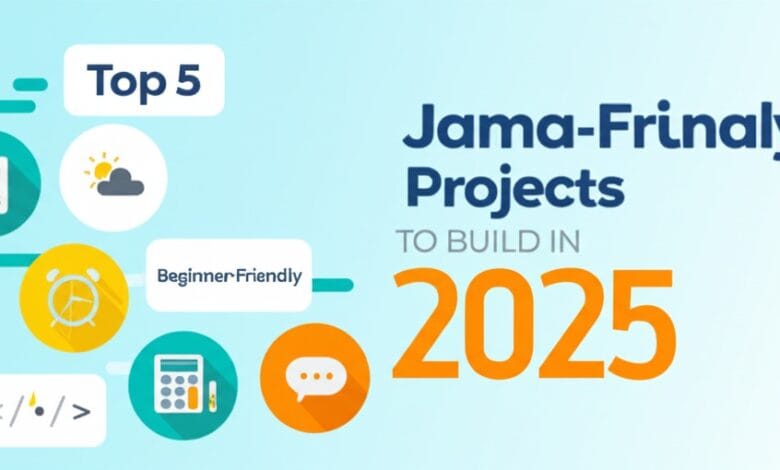
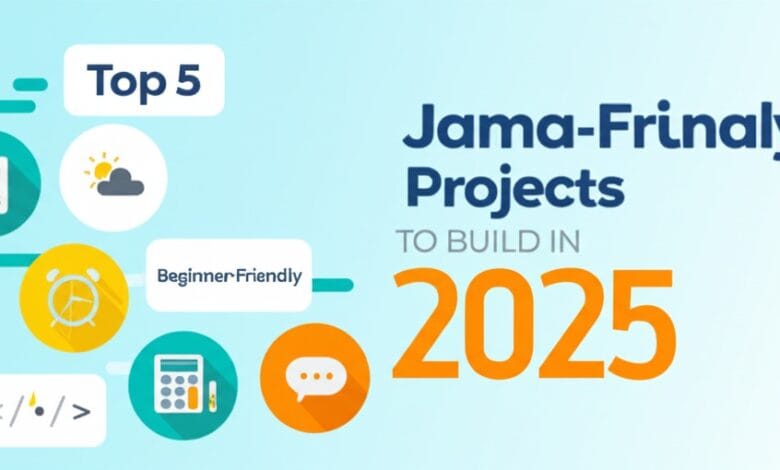
Summary: Learning JavaScript effectively involves hands-on practice. This article explores five beginner-friendly JavaScript projects ideal for building in 2025, covering fundamental concepts like DOM manipulation, event handling, APIs, and local storage. These projects provide practical experience, build confidence, and create tangible portfolio pieces for aspiring developers.
The best way to transition from JavaScript theory to practical skill is by building projects. This article outlines five excellent starter projects for 2025 designed to solidify core concepts and boost your confidence as a new developer. Building these projects will give you hands-on experience with essential web development techniques.
Here’s a quick overview of what we’ll cover:
- Introduction: Why project-based learning is crucial for JavaScript beginners.
- Key Benefits: What you gain from building these specific projects.
- The Top 5 Projects (Detailed):
- Interactive To-Do List
- Simple Weather App
- Digital Clock & Timer
- Basic Calculator
- Random Quote Generator
- Tips for Success: How to approach building these projects effectively.
- Next Steps: Where to go after mastering these beginner projects.
- FAQ: Answering common questions beginners have about JavaScript projects.
Why Bother Building JavaScript Projects?
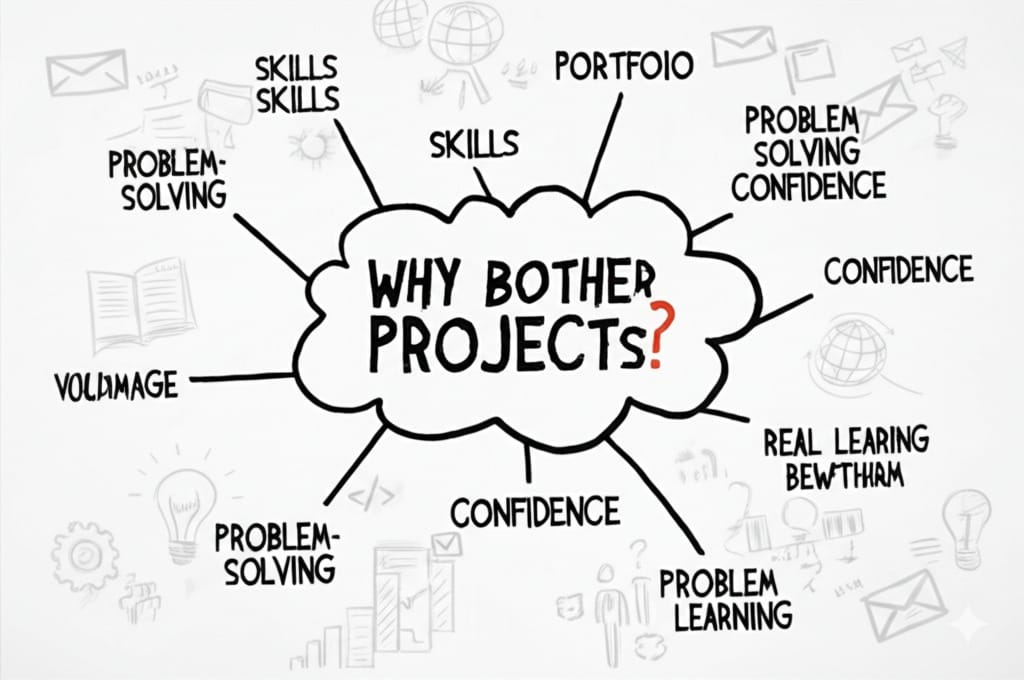
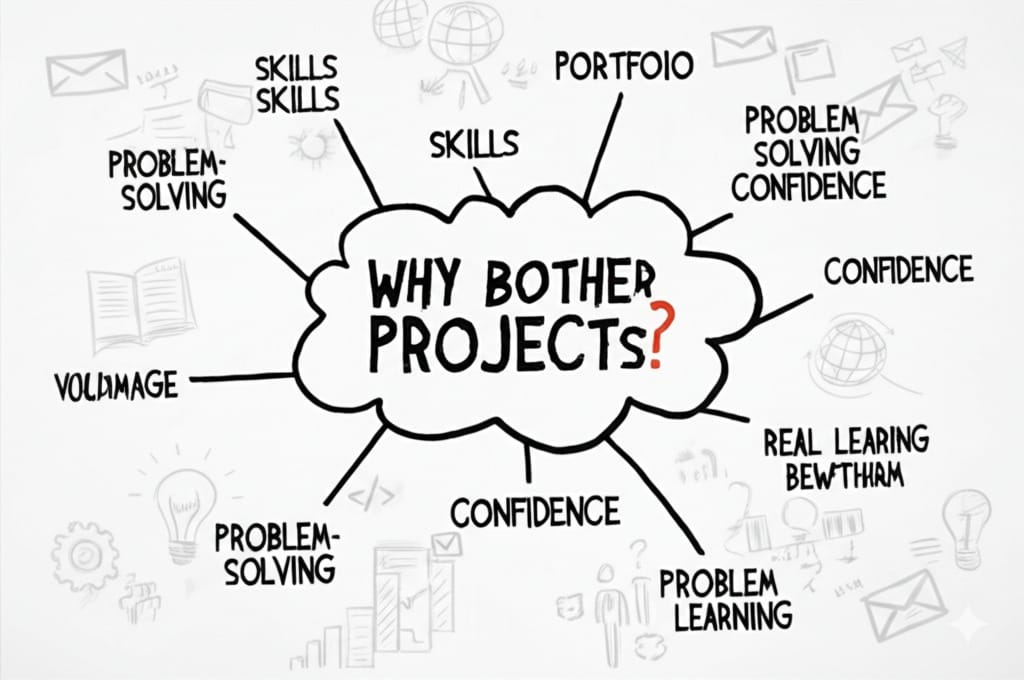
Reading documentation and watching tutorials is essential, but how do you truly learn JavaScript? By writing code. Building projects is the bridge between theoretical knowledge and practical application. It’s where concepts click, problems arise (and get solved!), and real learning happens.
Think of it like learning a musical instrument. You can read sheet music and understand music theory, but you won’t become proficient until you actually practice playing scales, chords, and eventually, full songs. JavaScript projects are your “songs.”
Here’s why investing time in building is non-negotiable for aspiring developers in 2025:
- Solidify Core Concepts: You’ll move beyond just knowing about DOM manipulation, event listeners, functions, and asynchronous code to actually using them purposefully. Abstract ideas become concrete tools.
- Develop Problem-Solving Skills: Every project presents unique challenges. Encountering bugs, figuring out logic flaws, and searching for solutions (a critical developer skill!) is inherent in the building process.
- Build a Portfolio: Even simple projects demonstrate your ability to code. These initial projects form the foundation of a portfolio you can show potential employers or collaborators.
- Boost Confidence: Successfully building something, no matter how small, is incredibly motivating. Each completed project proves to yourself that you can code.
- Discover Knowledge Gaps: You don’t know what you don’t know until you try to build something. Projects quickly highlight areas where your understanding is weak, guiding your future learning.
- Understand the Development Workflow: You’ll practice the cycle of planning, coding, testing, and debugging – the fundamental workflow of software development.
Project-based learning aligns with principles of active learning, which educational research suggests leads to deeper understanding and better retention compared to passive methods. (You can explore more about active learning strategies via resources like Vanderbilt University’s Center for Teaching: Vanderbilt CFT – Active Learning).
Now, let’s dive into five specific projects that offer a fantastic starting point for any JavaScript beginner in 2025.
Project 1: Interactive To-Do List
What is it?
A web application that allows users to add tasks, mark them as complete (often by clicking or striking through), and delete them. It’s a digital checklist managed entirely within the browser.
Why it’s Great for Beginners (What You’ll Learn)
This is often the quintessential first JavaScript project for good reason. It touches upon several fundamental concepts in a manageable scope:
- DOM Manipulation: You’ll learn how to dynamically create HTML elements (list items for each task), add them to the page, modify their content or style (e.g., striking through completed tasks), and remove them. This is the core of making web pages interactive.
- Event Handling: You need to listen for user actions – typing a new task, clicking the ‘add’ button, clicking a task to mark complete, clicking a ‘delete’ button. This introduces addEventListener and handling different event types (like submit, click).
- Working with Forms: Capturing user input from an input field is a common requirement. You’ll learn how to get the value entered by the user.
- Basic Data Management (Arrays): While not strictly necessary for the simplest version, managing tasks often involves storing them in a JavaScript array, iterating over it to display tasks, and updating it when tasks are added or removed.
- (Optional) Local Storage: To make the list persist even after the browser is closed, you can introduce localStorage. This teaches basic data persistence on the client-side.
Key Concepts Involved
- HTML Structure: A basic <ul> or <ol> list, an <input> field for adding tasks, and buttons (<button>).
- CSS Styling: Making it look presentable (though the focus is JS).
- JavaScript:
- document.getElementById() or document.querySelector() to select HTML elements.
- document.createElement() to create new HTML elements (e.g., <li>).
- .appendChild() or .prepend() to add elements to the DOM.
- .removeChild() to remove elements.
- .addEventListener() to listen for events (e.g., ‘click’, ‘submit’).
- .value property to get input from form fields.
- .textContent or .innerHTML to set the text of elements.
- .classList.toggle() or .style property to change appearance (e.g., line-through for completed).
- (Optional) localStorage.setItem(), localStorage.getItem(), JSON.stringify(), JSON.parse() for persistence.
Reference: For deep dives into DOM manipulation and events, the MDN Web Docs are invaluable:
* MDN: Introduction to the DOM
* MDN: Event reference
Potential Enhancements
- Add persistence using localStorage.
- Allow editing existing tasks.
- Add due dates or priority levels.
- Filter tasks (e.g., show only active or completed).
- Add animations for adding/removing tasks.
Project 2: Simple Weather App
What is it?
An application that takes a location (e.g., city name) as input from the user and displays the current weather conditions (temperature, description, humidity, wind speed, etc.) for that location.
Why it’s Great for Beginners (What You’ll Learn)
This project is a fantastic introduction to a crucial aspect of modern web development: interacting with external data sources (APIs).
- Fetching Data from an API: You’ll learn how to use the fetch API, JavaScript’s modern way to make network requests to get data from a server (in this case, a weather API provider).
- Asynchronous JavaScript: API requests take time. You’ll learn about Promises and potentially async/await syntax to handle these asynchronous operations gracefully, ensuring your app doesn’t freeze while waiting for data.
- Handling JSON Data: APIs typically return data in JSON (JavaScript Object Notation) format. You’ll learn how to parse this data and extract the specific pieces of information you need (like temperature, weather description).
- Error Handling: What happens if the user enters an invalid city name or the API service is down? You’ll learn basic error handling (e.g., using .catch() with Promises or try…catch with async/await) to manage these situations.
- Dynamic Content Display: Similar to the To-Do list, you’ll update the DOM, but this time with data retrieved from an external source.
Key Concepts Involved
- HTML Structure: An input field for location, a button to submit, and elements (<div>, <span>, <p>) to display the weather information.
- CSS Styling: To present the weather data clearly.
- JavaScript:
- DOM selection and manipulation (as in Project 1).
- Event handling (for the submit button).
- fetch() API for making network requests.
- Promises (.then(), .catch()) or async/await syntax.
- .json() method to parse the response body.
- Accessing properties of JavaScript objects (the parsed JSON data).
- Basic error handling (try…catch or .catch()).
- (API Key Management): Understanding that many APIs require an API key for authentication (important: don’t commit keys directly into public code!). For beginner projects, free, keyless APIs or free tiers of keyed APIs are common.
Reference:
* MDN: Fetch API
* MDN: Using Fetch
* MDN: Async/await
* Example Free Weather API: OpenWeatherMap (Free Tier) – Requires signup for an API key.
* Government Weather Data (More complex, but illustrates public data sources): National Weather Service API (weather.gov)
Potential Enhancements
- Use browser geolocation API to get weather for the user’s current location.
- Add icons representing the weather condition.
- Display a forecast for the next few days.
- Allow users to switch between Celsius and Fahrenheit.
- Improve error handling and user feedback (e.g., “City not found”).
- Add a loading indicator while fetching data.
Project 3: Digital Clock & Timer
What is it?
A simple web component that displays the current time, updating every second. Optionally, it can be extended to include a countdown timer or stopwatch functionality.
Why it’s Great for Beginners (What You’ll Learn)
This project focuses on working with dates, times, and timed events in JavaScript.
- JavaScript Date Object: You’ll learn how to create instances of the Date object and use its methods (getHours(), getMinutes(), getSeconds(), etc.) to get the current time components.
- Timed Functions (setInterval): The core of the clock is updating the display every second. You’ll use setInterval() to execute a function repeatedly at a specified interval (1000 milliseconds).
- DOM Updates: Continuously updating the displayed time on the webpage.
- Formatting: Handling single-digit numbers (e.g., displaying “07” instead of “7” for seconds) often requires basic string manipulation or conditional logic.
- (For Timer/Stopwatch) clearInterval, State Management: Managing the start/stop/reset state of a timer involves more complex logic and using clearInterval() to stop the timed updates.
Key Concepts Involved
- HTML Structure: A single element (e.g., a <div> or <p>) to display the time. Buttons if implementing timer/stopwatch functionality.
- CSS Styling: Basic styling for the clock display.
- JavaScript:
- new Date() to get the current date and time.
- Date object methods: .getHours(), .getMinutes(), .getSeconds(), .toLocaleTimeString() (useful for formatting).
- setInterval(callbackFunction, delayInMilliseconds) to run code repeatedly.
- clearInterval(intervalID) to stop setInterval.
- DOM selection and updating .textContent.
- String manipulation (e.g., padding numbers with leading zeros using .padStart()).
- (For Timer/Stopwatch) Variables to keep track of time elapsed or remaining, conditional logic (if/else) for state management.
Reference:
* MDN: Date Object
* MDN: setInterval()
* MDN: clearInterval()
Potential Enhancements
- Add AM/PM indicator.
- Allow users to choose between 12-hour and 24-hour formats.
- Implement a countdown timer: user sets duration, clicks start, time counts down. Play a sound when finished.
- Implement a stopwatch: start, stop, lap, reset functionality.
- Display the current date as well.
- Add theme options (e.g., light/dark mode).
Project 4: Basic Calculator
What is it?
A web-based simulation of a simple electronic calculator that can perform basic arithmetic operations (addition, subtraction, multiplication, division).
Why it’s Great for Beginners (What You’ll Learn)
This project heavily emphasizes logic, event handling for multiple elements, and managing application state.
- Handling Multiple Events: You’ll attach event listeners to many buttons (numbers 0-9, operators +, -, *, /, equals, clear). This often leads to more efficient ways of handling events (e.g., event delegation).
- User Interface Logic: Figuring out how to display numbers as they are clicked, how to store the first number when an operator is pressed, how to perform the calculation when ‘equals’ is clicked, and how to handle sequential operations or clearing the display.
- String Manipulation & Type Conversion: User input from buttons often comes in as strings. You’ll need to concatenate number strings for display, convert strings to numbers (parseInt(), parseFloat()) for calculations, and convert results back to strings for display.
- Basic Arithmetic Operations: Implementing the core math logic.
- State Management: Keeping track of the current number being entered, the previous number, the selected operator, and whether a calculation was just performed are key challenges that teach basic state management.
Key Concepts Involved
- HTML Structure: A display area (<input type=”text”> or <div>) and lots of <button> elements for numbers, operators, clear, and equals. Grid layout (CSS Grid or Flexbox) is useful here.
- CSS Styling: Making it look like a calculator.
- JavaScript:
- DOM selection (selecting all buttons might involve querySelectorAll).
- addEventListener (potentially using event delegation on the button container).
- Variables to store state (e.g., currentOperand, previousOperand, operator).
- String concatenation.
- parseInt(), parseFloat(), or Number() for type conversion.
- Basic arithmetic operators (+, -, *, /).
- Conditional logic (if/else or switch statements) to handle different button clicks (number, operator, equals, clear).
- Updating the display (.value or .textContent).
- Handling edge cases (e.g., division by zero, multiple decimal points).
Reference:
* MDN: Event Delegation (Useful for handling many buttons)
* MDN: Math Operators
* MDN: Number Conversion
Potential Enhancements
- Add keyboard support (allow typing numbers and operators).
- Implemented decimal point functionality correctly.
- Add memory functions (MC, MR, M+, M-).
- Include more advanced operations (square root, percentage).
- Handle calculation chaining (e.g., 5 + 3 * 2 = 11, respecting operator precedence, or simply calculating sequentially).
- Improve visual feedback (e.g., button press effects).
Related topic: Master CSS Functions: The Ultimate Cheat Sheet for Modern Web Design
Project 5: Random Quote Generator
What is it?
A simple application that displays a random quote (and optionally its author) each time the user clicks a button.
Why it’s Great for Beginners (What You’ll Learn)
This project reinforces DOM manipulation and event handling, and it can be implemented with either local data or an API, offering flexibility.
- Working with Arrays/Objects: If storing quotes locally, you’ll learn to structure data in arrays of objects (each object containing the quote text and author) and randomly select an item from the array.
- Basic Randomness (Math.random): Generating a random index to pick a quote from the array.
- DOM Manipulation: Updating the text content of elements on the page to display the new quote and author.
- Event Handling: Listening for a click on a “New Quote” button.
- (Optional) Fetching Data from an API: Similar to the Weather App, you could fetch quotes from a public quote API, reinforcing asynchronous JavaScript concepts (fetch, Promises or async/await).
Key Concepts Involved
- HTML Structure: Elements to display the quote (<blockquote> or <p>) and the author (<cite> or <p>), and a <button> to trigger fetching a new quote.
- CSS Styling: Basic presentation.
- JavaScript:
- DOM selection and updating .textContent or .innerHTML.
- addEventListener for the button click.
- If using local data:
- An array of objects storing quotes [{text: “…”, author: “…”}, …].
- Math.random() to generate a random number.
- Math.floor() to get a whole number index.
- Array indexing (myArray[randomIndex]) to select a quote.
- If using an API:
- fetch() API.
- Promises (.then(), .catch()) or async/await.
- JSON parsing (.json()).
- Accessing data from the API response object.
Reference:
* MDN: Math.random()
* MDN: Working with JSON
* Example Quote APIs (check terms of use): Type.fit API (simple, no key required), Quotable API
Potential Enhancements
- Add a “Tweet Quote” button that opens Twitter with the current quote pre-filled.
- Fetch quotes from an external API instead of a local array.
- Allow users to filter quotes by category or author (if the API supports it or if you categorize your local data).
- Animate the transition between quotes.
- Store the previously displayed quote to avoid showing the same one twice in a row.
Tips for Success When Building Your First Projects
Embarking on these projects is exciting, but it can also be challenging. Here’s how to approach them effectively:
- Start Simple: Don’t try to implement all potential enhancements at once. Get the core functionality working first. For the To-Do list, just get adding and displaying tasks working before worrying about deleting, completing, or local storage.
- Break It Down: Decompose the project into smaller, manageable steps. What’s the very first thing you need? (e.g., “Get the button element”). What’s next? (“Make something happen when the button is clicked”). Small victories build momentum.
- Plan (A Little): Sketch out the basic HTML structure. Think about what JavaScript needs to do. You don’t need a formal specification, but a rough plan helps. Pseudocode (writing out the logic steps in plain English) can be very useful.
- Use Developer Tools: Your browser’s developer tools (Console, Elements, Network tabs) are your best friends. Use console.log() liberally to check variable values, see if functions are running, and understand the flow of your code. Inspect the DOM to see if elements are being created correctly. Check the Network tab for API requests.
- Read Documentation: When you encounter a method or concept you don’t understand (like fetch or localStorage), go to the MDN Web Docs. It’s the definitive resource.
- Don’t Just Copy-Paste: While looking at tutorials or solutions is fine when you’re stuck, try to understand why the code works. Type it out yourself rather than copying and pasting. Experiment by changing parts of it to see what happens.
- Embrace Errors: Errors in the console aren’t failures; they are information. Read the error message carefully. It often tells you exactly where the problem is and what type of error occurred. Google the error message – chances are someone else has faced the same issue.
- Version Control (Git): Even for small projects, start using Git. Commit your code frequently with meaningful messages. It allows you to revert changes if you break something and is a fundamental skill for collaboration. Platforms like GitHub or GitLab offer free repositories.
- Be Patient: Learning takes time. You will get stuck. That’s part of the process. Take breaks, walk away, and come back with fresh eyes.
Beyond the Basics: What’s Next?
Once you’ve successfully built these beginner projects and feel comfortable with the core concepts, you can start exploring more advanced areas:
- More Complex Projects: Try building things like a simple blog (fetching posts from an API or local JSON), a memory game, a simple e-commerce product page (adding items to a cart), or a Pomodoro timer.
- JavaScript Frameworks/Libraries: Explore popular tools like React, Vue, or Angular. These provide structures and tools for building larger, more complex single-page applications (SPAs). Building the beginner projects in vanilla JS first provides a strong foundation for understanding what these frameworks do for you.
- Node.js: Learn server-side JavaScript with Node.js. This allows you to build full-stack applications, create your own APIs, and interact with databases.
- Advanced Concepts: Dive deeper into asynchronous JavaScript, functional programming concepts, modules, build tools (like Vite or Webpack), and testing.
- Contribute to Open Source: Find beginner-friendly issues on open-source projects to contribute to. It’s a great way to learn from experienced developers and gain real-world experience.
Conclusion
What’s the best way to learn JavaScript in 2025? By building things. The five projects outlined here – the To-Do List, Weather App, Digital Clock, Calculator, and Quote Generator – provide a structured path for beginners to apply fundamental concepts in practical ways. They cover DOM manipulation, event handling, APIs, asynchronous operations, and basic state management – skills essential for any aspiring web developer.
Don’t aim for perfection on your first try. Focus on learning, problem-solving, and the satisfaction of creating something functional. Each project you complete builds not only your technical skills but also your confidence and your portfolio. Start coding, embrace the challenges, and enjoy the journey of becoming a JavaScript developer!
Frequently Asked Questions (FAQ)
(Schema-ready Q&A format)
Q: What HTML and CSS knowledge do I need before starting these JavaScript projects?
A: You should have a solid understanding of basic HTML structure (tags like <div>, <p>, <ul>, <li>, <input>, <button>) and fundamental CSS concepts (selectors, properties like color, background-color, margin, padding, and basic layout techniques like Flexbox or Grid). You don’t need to be a CSS expert, but you should be comfortable creating the visual layout for these simple applications.
Q: How long should each of these beginner JavaScript projects take?
A: This varies greatly depending on your current skill level, how much time you dedicate, and how deep you go with enhancements. A focused beginner might complete the core functionality of each project in anywhere from a few hours to a couple of days. The key is not speed, but understanding. Don’t rush; focus on learning the concepts involved.
Q: What if I get stuck while building a project?
A: Getting stuck is normal! First, try to debug using console.log() and your browser’s developer tools. Re-read the relevant documentation (like MDN). Break the problem down into smaller pieces. Try explaining the problem out loud (rubber duck debugging). Search for specific error messages or conceptual problems on sites like Stack Overflow. If you’re truly blocked, look at a tutorial or solution for that specific part, but try to understand it before implementing it.
Q: Do I need to use a JavaScript framework like React or Vue for these projects?
A: No, absolutely not! These projects are designed to be built with vanilla JavaScript (plain JS without frameworks). The goal is to learn the fundamental language features and web APIs (like the DOM and Fetch). Learning these basics first will make understanding frameworks much easier later on.
Q: Where can I find free APIs to use for projects like the Weather App or Quote Generator?
A: There are many free APIs available for practice. For weather, OpenWeatherMap has a popular free tier (requires an API key). For quotes, APIs like Type.fit or Quotable are often used. You can also search GitHub or sites like Public APIs for lists of free APIs covering various topics (like books, movies, space data, etc.). Always check the API documentation for usage limits and attribution requirements.
Q: How do I showcase these projects once I’ve built them?
A: The best way is to deploy them online! Services like GitHub Pages, Netlify, or Vercel offer free hosting for static websites (HTML, CSS, client-side JS). You can put your code on GitHub and link the live deployed versions from your repository’s README file or your personal portfolio website. This allows potential employers or collaborators to easily see your work in action.
Q: Is it okay if my project code isn’t perfect or looks different from examples?
A: Yes, completely okay! Especially as a beginner. The primary goal is learning and making it work. Code quality, optimization, and advanced patterns come with experience. Focus on functionality and understanding first. As you learn more, you can always revisit and refactor your earlier projects.