How Do Computers Work? Computer Science Explained (The Fun Way)
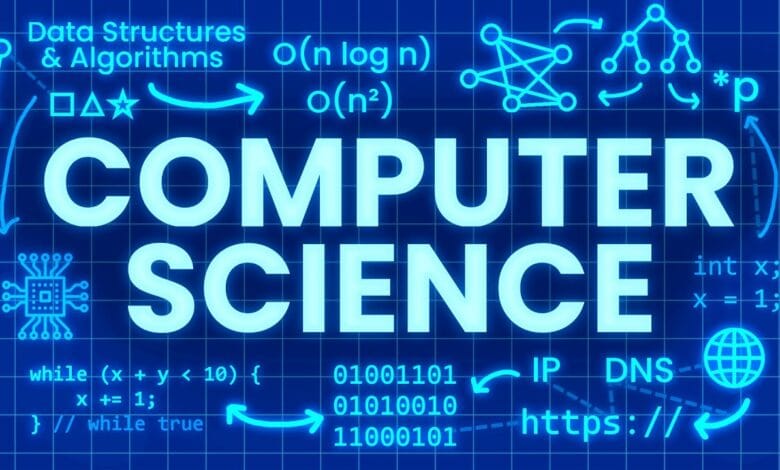
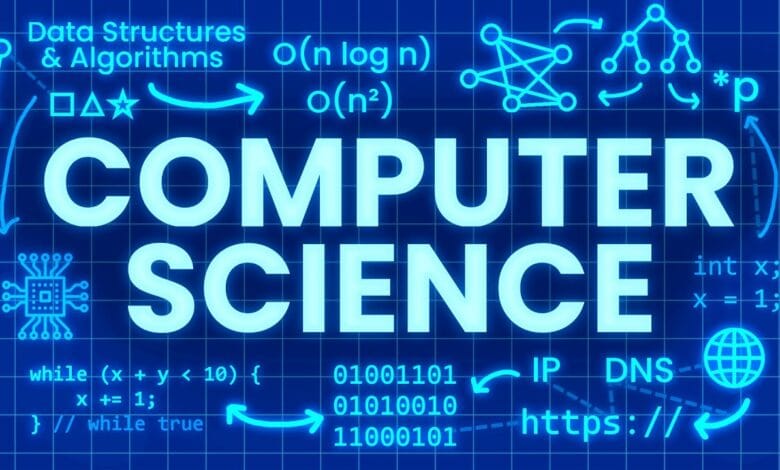
Introduction:
Your Quick Guide to Computer Science
Ever wondered how computers actually work? At their core, they process information using billions of tiny switches (transistors) representing data as 0s and 1s, following programmed instructions to perform tasks. This guide breaks down the essentials of computer science – from hardware basics and binary code to programming, data structures, the internet, and even a touch of machine learning – all explained simply (and maybe with a few memes in mind) in about 15 minutes. Perfect as a refresher or a first look!
Key Points:
- Hardware Basics: What CPUs, transistors, and binary code are.
- Data Representation: Understanding bits, bytes, hexadecimal, and ASCII.
- Core Concepts: How logic gates, operating systems, and memory (RAM) function.
- Programming Essentials: Different languages, compilers vs. interpreters, variables, and data types.
- Organizing Data: Exploring arrays, linked lists, hash maps, trees, and graphs.
- Algorithms & Logic: Functions, recursion, efficiency (Big O notation).
- Web & Networking: The basics of the Internet, WWW, HTTP, databases (SQL).
- A Glimpse Beyond: Introduction to Machine Learning and basic security concepts.
How Computer Hardware Works: The Building Blocks
Let’s peek inside your PC and see what makes it tick.
The CPU and Transistors: The Brain’s Switches
Inside your computer is a Central Processing Unit (CPU). Think of it as the brain. It’s essentially a piece of silicon packed with billions of microscopic switches called transistors.
- These transistors can be either ON or OFF, depending on electricity flow.
- This gives us two states: 1 (ON) and 0 (OFF).
Bits, Bytes, and Binary: The Language of Computers
- Bit: The value (1 or 0) at a single transistor is called a “bit”. One bit isn’t very useful alone.
- Byte: Group 8 bits together, and you get a “byte”. A byte can represent 256 different combinations of 0s and 1s (2^8).
- Binary: This system of 0s and 1s is called “binary”. We use it to store information. Each bit position represents a power of 2. For example, 01000101 means (0128) + (164) + (032) + (016) + (08) + (14) + (02) + (11) = 69.
Hexadecimal: Making Binary Readable
Binary is clunky for humans. Hexadecimal (often shown with 0x) helps:
- It groups binary bits into sets of four.
- Four bits can represent values 0-15.
- Hexadecimal uses digits 0-9 and letters a-f to represent these 16 values.
- This makes long binary strings much shorter and easier to read.
Logic Gates and Boolean Algebra: Making Decisions
Using transistors, we create logic gates. These are circuits that perform logical operations:
- Think of a lightbulb with switches: an AND gate only turns the light on if Switch A AND Switch B are ON.
- Combining gates allows for complex calculations based on Boolean algebra, the math of binary logic (TRUE/FALSE or 1/0).
ASCII: Turning Numbers into Letters
How does typing ‘A’ show ‘A’ on screen? We use character encodings like ASCII:
- Each character (letter, number, symbol) is assigned a unique binary number.
- When you press ‘A’, the keyboard sends its binary code (e.g., 01000001).
- The computer recognizes this code and displays ‘A’.
The Operating System and Processing
Hardware needs instructions and management. That’s where the OS and processing cycle come in.
Operating System Kernel: The Traffic Cop
An Operating System (OS) kernel (like Windows, Linux, macOS) acts as a bridge:
- It sits between the hardware (CPU, RAM, devices) and the software applications.
- It manages how everything works together, using things like device drivers to talk to specific hardware.
Machine Code: The CPU’s Native Tongue
Input devices let you give commands, but at the lowest level, computers only understand machine code:
- This is raw binary code directly telling the CPU what operation to perform and what data to use.
RAM: The Computer’s Short-Term Memory
The CPU can process instructions but can’t store much data itself. It needs Random Access Memory (RAM):
- Imagine RAM as a grid of boxes.
- Each box holds one byte (data or instruction) and has a unique address.
- The CPU uses this address to quickly access any box.
The Fetch-Execute Cycle: How Work Gets Done
The CPU constantly repeats a basic cycle to run programs:
- Fetch: Get the next instruction from RAM using its address.
- Decode: Figure out what the instruction means.
- Execute: Perform the operation (e.g., add two numbers).
- Store: Write the result back to RAM or a CPU register.
This happens billions of times per second!
CPU Speed and Cores: Faster and Parallel Processing
- Clock Speed (GHz): A clock generator synchronizes these cycles. Higher Gigahertz (GHz) means more cycles per second (faster processing). Overclocking pushes this speed, but can generate excess heat.
- Cores: Modern CPUs have multiple cores. Each core can execute instructions independently and in parallel (at the same time).
- Threads: Each core can often handle multiple threads, allowing it to switch between different instruction sequences concurrently (very quickly).
Shell: Talking to the Kernel
The kernel is usually wrapped in a shell. This is a program (like Command Prompt or Terminal) that lets users interact with the kernel using text commands in a command-line interface (CLI).
Understanding Programming Basics
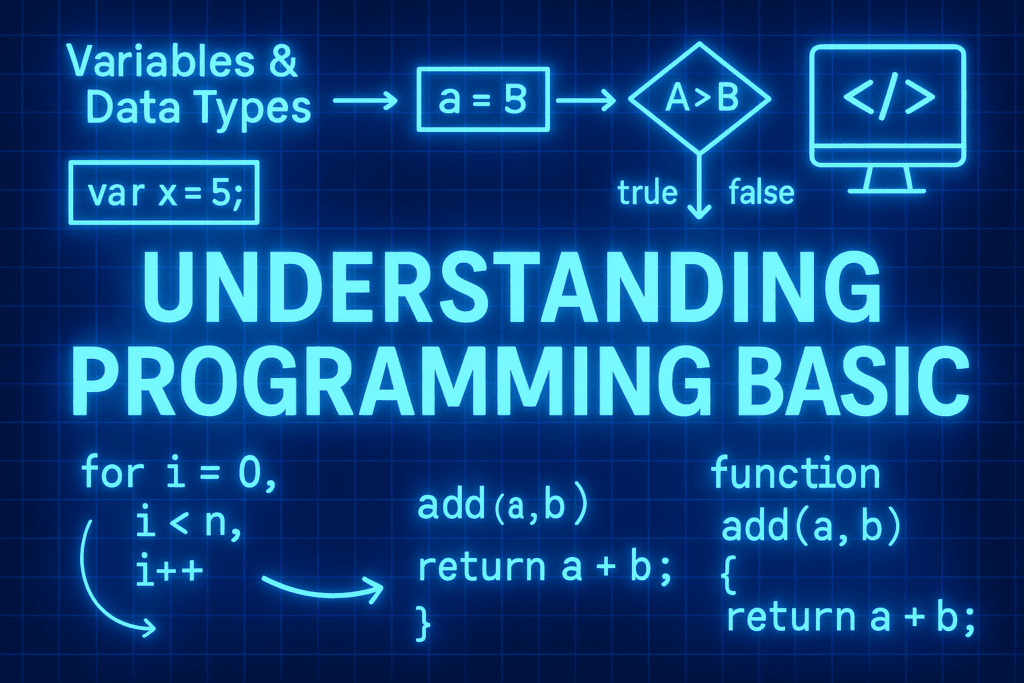
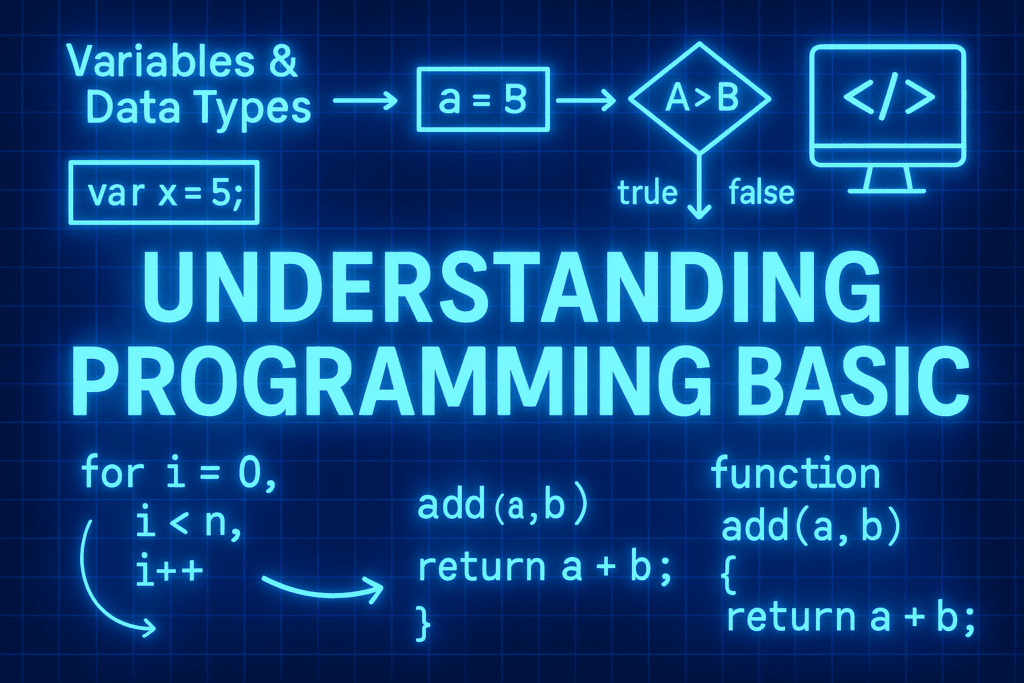
Humans don’t write machine code directly. We use programming languages.
Programming Languages: Bridging Human and Machine
Programming languages use abstraction to let us write code that resembles human language. This code then needs to be converted to machine code. There are two main ways:
- Interpreters (e.g., Python): Read and execute the source code line by line.
- Compilers (e.g., C, Go): Translate the entire program into a machine code file first, which the CPU then executes.
Variables & Data Types: Storing Information
Variables are names given to storage locations for data. The type of data determines how it’s stored and used:
- Text: char (single character), string (sequence of characters).
- Whole Numbers: int (integer), can be signed (positive/negative) or unsigned (positive only).
- Decimal Numbers: float (floating-point). They use scientific notation (number x base^exponent) and binary fractions. This can sometimes lead to rounding errors (e.g., 0.1 + 0.2 might not be exactly 0.3).
- Larger Numbers: long (bigger integer range), double (bigger float range/precision). Use twice the memory.
- Boolean: bool (True or False).
Some languages (like Python) figure out the type automatically (dynamic typing). Others (like C) require you to declare the type explicitly (static typing).
Pointers: Variables Holding Addresses
- A variable’s value is stored at a specific memory address.
- Pointers are special variables that store the memory address of another variable. (In C, &variable gets the address).
- Pointer Arithmetic: Since addresses are numbers, you can add/subtract to move between adjacent memory locations (useful but dangerous!).
Memory Management: Keeping Track of Space
- Manual (e.g., C): Programmers must explicitly allocate memory when needed and free it when done. This happens in the heap, a flexible memory area. Mistakes are easy:
- Segmentation Fault: Accessing memory you shouldn’t.
- Memory Leak: Forgetting to free memory, making it unusable and slowing/crashing the program.
- Automatic (e.g., Python, Java): A garbage collector automatically finds and frees up memory that’s no longer being used. Much safer, less control.
Organizing Data: Structures and Algorithms
How we store data greatly affects how efficiently we can use it.
What are Data Structures?
A data structure is a specific way of organizing and storing data to perform operations on it efficiently.
Arrays: Ordered Lists in Memory
- An array stores a list of items of the same data type in a contiguous block of memory.
- Each item has a numerical index (usually starting at 0).
- Pros: Very fast access to any element using its index (thanks to pointer arithmetic).
- Cons: Size is often fixed at creation. Adding more items than capacity requires creating a new, larger array. Wasted space if not full.
Linked Lists: Flexible Chains
- A linked list uses nodes. Each node contains:
- The data value.
- A pointer to the next node in the sequence.
- Pros: Nodes can be anywhere in memory. Can easily grow, shrink, add, remove, and reorder nodes by changing pointers.
- Cons: To find an element (especially the last), you might have to traverse the entire list from the beginning. Slower access than arrays.
Stacks & Queues: Orderly Data Handling
Both can be built using arrays or linked lists:
- Stack: Last-In, First-Out (LIFO). Like a stack of pancakes. You push (add) to the top and pop (remove) from the top.
- Queue: First-In, First-Out (FIFO). Like a waiting line. You enqueue (add) at the back and dequeue (remove) from the front.
Hash Maps (Dictionaries): Key-Value Pairs
- A hash map (or dictionary) stores key-value pairs.
- It uses a hash function to convert a key into an array index where the value is stored.
- Pros: Extremely fast lookups, insertions, and deletions (on average) using the key. Keys can be descriptive (not just numbers).
- Cons: Collisions can happen when different keys hash to the same index. Strategies exist to handle this (like using linked lists at that index), which can slightly slow down access in those cases.
Graphs: Representing Connections
- Imagine linked list nodes where any node can point to any other node. That’s a graph.
- Nodes (or vertices) are connected by edges.
- Edges can be:
- Directed (A -> B) or Undirected (A <-> B).
- Weighted (carrying a value like distance or cost).
- Uses: Social networks, mapping (finding shortest paths), network analysis.
- Searching:
- Breadth-First Search (BFS): Explores layer by layer from the start node. Finds the shortest path in unweighted graphs.
- Depth-First Search (DFS): Explores one path as far as possible, then backtracks and tries another.
Trees: Hierarchical Structures
- A tree is a special type of graph with no cycles, where any two nodes are connected by exactly one path. Represents hierarchy.
- Starts at a root node.
- Branches into subtrees.
- Nodes with children are parent nodes.
- Nodes with no children are leaf nodes.
- Binary Tree: Each node has at most two children (left and right).
- Binary Search Tree (BST): A binary tree where for any node:
- All values in the left subtree are smaller.
- All values in the right subtree are larger.
- Pros: Very fast searching, insertion, and deletion (if balanced). To find a value, compare with the current node: go left if smaller, go right if larger, repeat.
Algorithms and Logic Flow
Data structures are useful, but we need instructions to work with them.
What is an Algorithm?
An algorithm is a step-by-step set of instructions designed to solve a specific problem or perform a task.
Functions: Reusable Code Blocks
- A function (or method) bundles a sequence of instructions.
- It can take inputs (arguments/parameters).
- It performs actions.
- It can return an output.
- You can call a function by its name, potentially passing different arguments each time, making code reusable.
The Call Stack: Managing Function Calls
When a function is called, it’s pushed onto the call stack (a stack data structure in memory used for execution). When a function finishes, it’s popped off. This manages the flow, especially when functions call other functions.
Booleans, Conditionals, and Loops: Controlling Flow
- Boolean Logic: Comparing values (>, ==, !=) and using logical operators (AND, OR, NOT) results in a Boolean value (True or False).
- Conditional Statements: Execute code based on Boolean conditions:
- if condition is true: do this
- else: do that
- elif (else if): check another condition
- Loops: Repeat blocks of code:
- while loop: Repeats while a condition remains True.
- for loop: Repeats for a specific number of times or iterates over each item in a sequence (like an array or list).
Recursion: Functions Calling Themselves
- A function that calls itself is recursive.
- Useful for problems breakable into smaller, self-similar subproblems (e.g., calculating factorial: 5! = 5 * 4!).
- Danger: Must have a base case – a condition to stop the recursion. Otherwise, it calls itself forever, filling the call stack and causing a stack overflow crash.
- Memoization: To optimize recursion (and other computations), store the results of expensive function calls and return the cached result if the same inputs occur again. Avoids re-computation.
Time Complexity & Big O Notation: Measuring Efficiency
How good is an algorithm? We measure its time complexity (how runtime grows with input size) and space complexity (how memory usage grows).
- Big O Notation describes the growth rate as input size approaches infinity.
- O(1): Constant time (e.g., accessing an array element by index). Fastest.
- O(log n): Logarithmic time (e.g., binary search in a sorted array). Very fast.
- O(n): Linear time (e.g., iterating through an array once). Good.
- O(n log n): Log-linear time (e.g., efficient sorting algorithms). Quite good.
- O(n^2): Quadratic time (e.g., nested loops comparing all pairs in an array). Okay for small inputs.
- O(2^n): Exponential time (e.g., some recursive solutions without memoization). Slows down dramatically.
- O(n!): Factorial time. Extremely slow.
Focus is on the trend, constants and lower-order terms are dropped (e.g., O(2n + 3) becomes O(n)).
Algorithm Approaches: Strategies for Problem Solving
- Brute Force: Try every possible solution. Simple, but often inefficient. (e.g., checking every item in a list).
- Divide and Conquer: Break the problem into smaller, independent subproblems, solve them, and combine the results. (e.g., Binary Search, Merge Sort).
- Greedy: Make the locally optimal choice at each step hoping it leads to a global optimum. (Not always works).
- Dynamic Programming: Break down into overlapping subproblems, solve each subproblem once, store the result (memoization/tabulation), and reuse it.
Programming Paradigms: Styles of Coding
Different ways to structure code to solve problems:
- Imperative: Tells the computer how to accomplish a task with step-by-step instructions that change the program’s state. (e.g., using loops and assignments).
- Object-Oriented Programming (OOP): An extension of imperative. Organizes code around objects, which bundle data (properties) and behavior (methods).
- Class: A blueprint for creating objects.
- Encapsulation: Bundling data and methods within a class.
- Inheritance: Creating new classes (subclasses) that reuse properties/methods from existing classes (superclasses), allowing extension or modification.
- Polymorphism: Objects of different classes can be treated as objects of a common superclass, but respond differently to the same method call (e.g., a Dog object and Cat object both have a makeSound() method, but produce different sounds).
- Object-Oriented Programming (OOP): An extension of imperative. Organizes code around objects, which bundle data (properties) and behavior (methods).
- Declarative: Tells the computer what result you want, without specifying how to get it. (e.g., SQL queries, HTML structure).
Read also: Why Your AI Buddy Gets Dumb Sometimes: Context Windows Explained
A Glimpse Beyond: Machine Learning
What if you can’t explicitly code the rules?
Machine Learning: Learning from Data
Machine Learning (ML) teaches computers to perform tasks without being explicitly programmed for them. Example: Recognizing a bee in images.
- Data: Collect lots of labeled data (images marked as ‘bee’ or ‘not bee’). Split into training data and test data.
- Algorithm: Choose a model that can learn (e.g., a neural network with adjustable ‘weights’).
- Training: Feed the training data into the algorithm. The model adjusts its internal parameters (weights) to better match the labels.
- Evaluation: Use the test data (which the model hasn’t seen) to check the model’s accuracy.
- Improvement: If inaccurate, use techniques (like comparing output to the correct answer via an error function) to further tweak the parameters, minimizing the error.
Related: What is Machine Learning? A Simple Explanation for Beginners
Connecting to the World: The Internet and Web
Computers talk to each other globally.
The Internet: The Global Network Hardware
- The Internet is a vast, physical network connecting computers worldwide.
- Uses undersea cables, satellites, routers, and Internet Service Providers (ISPs) to link everything.
Internet Protocols: The Rules of Communication
Computers use the Internet Protocol (IP) Suite to communicate:
- IP Address: Every device on the network gets a unique numerical label (like a postal address).
- Transmission Control Protocol (TCP): Breaks messages into smaller packets, sends them across the network (possibly via different routes), and reassembles them at the destination. Ensures reliable delivery. Packet loss occurs when packets get lost (common with bad connections).
World Wide Web (WWW): The Information Space
- If the Internet is the hardware/roads, the Web is the software/information running on it (websites, applications).
- Accessed using a web browser.
How You Access a Website
- URL: You type a website address (Uniform Resource Locator) into your browser.
- DNS Lookup: The browser asks the Domain Name System (DNS) – the internet’s phonebook – to translate the human-readable domain name (e.g., www.google.com) into the server’s numerical IP address.
- TCP Connection: Your browser establishes a TCP connection with the server at that IP address.
- HTTP Request: Your browser (the client) sends a Hypertext Transfer Protocol (HTTP) request to the server (e.g., “GET me the homepage”).
- HTTP Response: The server processes the request and sends back an HTTP response, usually containing the website’s content (or an error).
Website Content: HTML, CSS, JavaScript
Websites are typically built with:
- HTML (HyperText Markup Language): Defines the structure and content (text, links, images, buttons).
- CSS (Cascading Style Sheets): Controls the presentation and visual styling (colors, fonts, layout).
- JavaScript: Adds interactivity and dynamic behavior (animations, form validation, fetching data).
HTTP Status Codes: Server Feedback
Every HTTP response includes a status code:
- 200 OK: Request successful.
- 301 Moved Permanently: Page moved.
- 404 Not Found: The requested resource doesn’t exist. (Most famous error!)
- 403 Forbidden: You don’t have permission to access this.
- 500 Internal Server Error: Something went wrong on the server side.
HTTP Methods: Types of Requests
HTTP requests have methods indicating the desired action:
- GET: Retrieve data (e.g., load a webpage).
- POST: Submit data to be processed (e.g., submit a form).
- PUT: Update an existing resource.
- DELETE: Remove a resource.
These methods are often used by APIs (Application Programming Interfaces), which allow different software applications to communicate and exchange data (e.g., a web app talking to a database).
Databases and Security
Where does all the data live, and how do we keep it safe?
Relational Databases: Structured Data Storage
- The most common type is a relational database.
- Stores data in tables (like spreadsheets).
- Columns: Represent different attributes (e.g., UserID, UserName, Email).
- Rows: Represent individual records (e.g., one user’s data).
- Primary Key: A unique identifier for each row in a table (e.g., UserID).
- Foreign Key: A column that refers to the Primary Key of another table, creating a relationship (e.g., an Orders table might have a UserID foreign key linking to the Users table).
SQL: Talking to Databases
Structured Query Language (SQL) is used to manage and query relational databases.
- SELECT column1, column2 FROM table WHERE condition;: Retrieve data.
- INSERT INTO table (column1) VALUES (value1);: Add data.
- UPDATE table SET column1 = value1 WHERE condition;: Modify data.
- DELETE FROM table WHERE condition;: Remove data.
- JOIN: Combines rows from two or more tables based on a related column (like a foreign key).
Caution: A poorly written SQL command (especially DELETE without a WHERE clause) can wipe out entire datasets!
SQL Injection Attacks: A Common Vulnerability
A major security risk for websites using databases:
- Login Example: A website might use SQL like: SELECT * FROM users WHERE username=’USER_INPUT’ AND password=’PASSWORD_INPUT’;
- The Attack: A hacker enters malicious input, like typing ‘ OR ‘1’=’1 into the username field.
- The Result: The SQL query might become SELECT * FROM users WHERE username=” OR ‘1’=’1′ — AND password=’…’
- The Problem: ‘1’=’1′ is always true, and — comments out the rest. The query now effectively asks “Find users where the username is empty OR true is true”. This bypasses the password check, potentially granting access.
Related: What’s This MCP Thing Everyone Might Start Talking About?
Frequently Asked Questions (FAQ)
Q1: What is the difference between hardware and software?
A: Hardware refers to the physical components of a computer you can touch (like the CPU, RAM, monitor, keyboard). Software refers to the programs and instructions that run on the hardware (like the operating system, web browser, games).
Q2: How does a CPU actually process instructions?
A: The CPU follows a Fetch-Execute Cycle: It fetches an instruction from memory (RAM), decodes what the instruction means, executes the operation, and stores the result. This cycle repeats billions of times per second.
Q3: What is binary code and why do computers use it?
A: Binary code is a system using only two digits, 0 and 1, to represent information. Computers use it because their fundamental components (transistors) have two states: ON (1) and OFF (0), making it easy to represent and manipulate data electronically.
Q4: Why are there so many different programming languages?
A: Different languages are designed for different purposes and offer different levels of abstraction, performance, and features. Some (like C) give low-level control, while others (like Python) prioritize ease of use. Some are compiled for speed, others interpreted for flexibility.
Q5: What’s the main purpose of using data structures?
A: Data structures provide efficient ways to organize, store, and retrieve data. Choosing the right structure (like an array for fast lookups by index, or a linked list for easy insertions/deletions) can significantly impact a program’s performance and complexity.
Q6: How does the Internet let me view a website?
A: When you enter a URL, your browser uses DNS to find the website server’s IP address. It then connects via TCP and sends an HTTP request. The server sends back an HTTP response containing the website’s HTML, CSS, and JavaScript files, which your browser renders into the page you see.
Computer Science resource links from authoritative and educational platforms, categorized for clarity:
Learning & Self-Study
- Teach Yourself Computer Science
- Comprehensive guide with free textbooks, video lectures, and project-based learning (e.g., Nand2Tetris for hardware/software integration) 2.
- Key Topics: Algorithms, Operating Systems, Networking, Databases.
- Link
- MIT OpenCourseWare (Computer Science)
- Free access to MIT course materials, including lectures and assignments 5.
- Link
- Open Textbook Library (Computer Science)
- Free, peer-reviewed textbooks on programming, data structures, and more 5.
- Link
Coding Practice & Problem-Solving
- Kattis
- Archive of programming challenges to sharpen algorithmic skills 7.
- Link
- Project Euler
- Mathematical/computer programming problems requiring creative solutions 7.
- Link
- Ted-Ed: Think Like a Coder
- Interactive video series teaching computational thinking through storytelling 7.
- Link
Teaching & Classroom Resources
- Teach Computing Curriculum
- Lesson plans, slides, and assessments for K-12 educators, aligned with UK standards 10.
- Link
- CS Unplugged
- Offline activities (games, puzzles) to teach CS concepts without computers 9.
- Link
- CSTA Resources Library
- Lesson plans, webinars, and equity-focused teaching tools for K-12 educators 4.
- Link
Research & Academic Tools
- ACM Digital Library & IEEE Xplore
- LibKey Nomad
- Browser extension for instant access to full-text academic articles 3.
- Link
Career & Industry Insights
- Amazon Future Engineer Career Tours
- Virtual field trips exploring tech careers at Amazon 4.
- Link
Open-Source & Community Resources
- FreeBASIC Compiler
- Open-source BASIC compiler for Windows, DOS, and Linux 7.
- Link
- GitHub Education
- Free developer tools and resources for students/educators (not listed in sources but highly recommended).